Spring Framework Overview
Goal of the Spring Framework
Spring Framework is a Java platform that provides comprehensive infrastructure support for developing Java applications.
- Spring handles the infrastructure (plumbing)
- so you can focus on your application (solving domain problem)
Spring makes it easy to create Java enterprise applications. It provides everything you need to embrace the Java language in an enterprise environment, and with the flexibility to create many kinds of architectures depending on an application’s needs. Spring is the de-facto most popular application development framework for enterprise Java.
Here some core modules of the Spring framework:
- Spring Context: For Dependency Injection (DI)
- Spring AOP: provides an AOP Alliance-compliant aspect-oriented programming implementation allowing you to define, for example, method-interceptors and pointcuts to cleanly decouple code implementing functionality that should logically speaking be separated.
- Spring DAO: The Data Access Object (DAO) support in Spring is aimed at making it easy to work with data access technologies like JDBC, Hibernate, JPA or JDO in a consistent way. This allows one to switch between the aforementioned persistence technologies fairly easily and it also allows one to code without worrying about catching exceptions that are specific to each technology.
- Spring JDBC: provides a JDBC-abstraction layer that removes the need to do tedious JDBC coding and parsing of database-vendor specific error codes.
- Spring ORM: provides integration layers for popular object-relational mapping APIs, including JPA, Hibernate, and myBatis.
- Spring Web module: provides basic web-oriented integration features, such as multipart file-upload functionality, the initialization of the IoC container using servlet listeners and a web-oriented application context. It also contains the web related parts of Spring's remoting support.
For you that strong in heart: check the structure and source codes of Spring Framework in github.
What Spring Framework Can Do For You?
The Spring Framework is divided into modules. Applications can choose which modules they need. At the heart are the modules of the core container, including a configuration model and a dependency injection mechanism. Beyond that, the Spring Framework provides foundational support for different application architectures, including messaging, transactional data and persistence, and web.
Spring core support are:
- Application Configuration
- Enterprise Integration
- Testing
- Data Access
Let's take a look one by one:
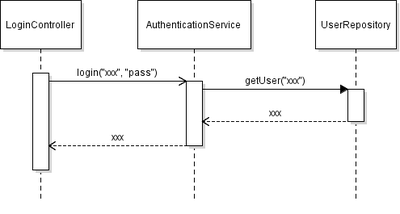
Login Sequence
Application Configuration
A typical application system consists of several parts working together to carry out a use case. Let's check following example, a login sequence.
Spring provides support for assembling such an application system from it's parts
- Parts do not worry about finding each other
- Any part can easily be swapped out
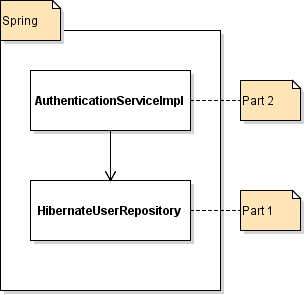
AuthenticationServiceImpl with Hibernate
Let's check two interfaces:
- UserRepository (part 1)
- AuthenticationService (part 2)
public interface UserRepository {
// interface members here
}
public interface AuthenticationService {
// interface members here
}
All the parts just POJOs (Plain Old Java Objects)
public class HibernateUserRepository
implements UserRepository {
}
public class AuthenticationServiceImpl
implements AuthenticationService {
private UserRepository userRepository;
/**
* @param userRepository the userRepository to set
*/
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
HibernateUserRepository implements UserRepository interface.
AuthenticationServiceImpl implements AuthenticationService.
Without Spring, we need to explicitly declare and set our parts:
HibernateUserRepository userRepository = new HibernateUserRepository();
AuthenticationServiceImpl authenticationService = new AuthenticationServiceImpl();
authenticationService.setUserRepository(userRepository);
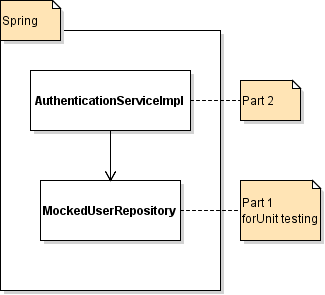
AuthenticationServiceImpl with Mocked Repository
But Spring is the one who done the plumbing for us, so we need no worry on how to assemble the AuthenticationService. Now, when we change the parts:
public class MockedUserRepository
implements UserRepository {
}
MockedUserRepository implements UserRepository interface.
Depends on how we configure, Spring will enable us to swith UserRepository implementation to MockedUserRepository. The idea is based on setUserRepository(UserRepository userRepository). As long us the type is UserRepository (which is applicable for both HibernateUserRepository and MockedUserRepository), then AuthenticationServiceImpl will accept it. This is can be done in Spring configuration.
UserRepository and AuthenticationService are service interfaces. Depends on these services we able to hide the complexity of implementation and in the same time allowing us for swapping out implementation.
If not using Spring plumbing via configuration, we need to do it manually:
MockedUserRepository userRepository = new MockedUserRepository();
AuthenticationServiceImpl authenticationService = new AuthenticationServiceImpl();
authenticationService.setUserRepository(userRepository);
but those codes are rigid, on the prospect on switching UserRepository implementation, we need to completely change the code.
Enterprise Integration
Enterprise applications do not work in isolation. They require enterprise resources and services, such as:
- Database transactions
- Security
- Messaging
- Remote access
- Caching
- etc...
Spring will help you to integrate powerful enterprise services into your application, while keeping your application code simple and testable.
Testing
Manual tests are expensive, in the sense of it's not scale. Need to be repeated each time the source code changes. And the worse part is, it's prone to errors. So, automated testing is essential. And the good news is, Spring enable unit testability by design. Spring decouples object from their environments, making it easier to test each piece of our application in isolation.
As in our example, we can do unit tests for our HibernateUserRepository and even AuthenticationServiceImpl. We also able to mock userRepository in AuthenticationServiceImpl.
Spring also provides system testing support, to help us to test all pieces together. This example show on how to enable Unit Testing in Spring:
import static junit.framework.Assert.assertEquals;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
public class AuthenticationServiceImplTest {
private AuthenticationServiceImpl authenticationService;
@Before
public void setUp() {
UserRepository userRepository = new MockedUserRepository();
authenticationService = new AuthenticationServiceImpl();
authenticationService.setUserRepository(userRepository);
}
@Test
public void login() {
User user = authenticationService.login("xxx", "pass");
assertEquals(user.getUserName(), "xxx");
}
}
Data Access
Most enterprise applications access and storing data. Majority of them, still using relational database to carry out business functions and to enforce business rules. Lately, peoples start moving to non-relational databases. Both are supported by Spring.
Spring makes data access easier to do effectively by:
- Manages resources for you
- Provides API helpers
- Supports all major data access technologies: JDBC, Hibernate, JPA, MyBatis, etc..., etc... (you name it)
Please check Spring Data.
Besides all those core supports, Spring also support:
- Web application development. Spring have Spring MVC and Web Flow, but also supporting major web framework like JSF and Struts. Spring also enable you to build web service with ease.
- Enterprise application development. Spring allow you to integrating messaging infrastructures (Spring Integration), securing services and providing object access control (Spring Security), Tasks and Job Scheduling (Spring Batch), and many enterprise grade services plumbing
- Cloud application development. Spring Cloud provides tools for developers to quickly build some of the common patterns in distributed systems.
And many more projects you can check in Spring projects website.