SpringFox Bean Validators for Swagger Documentation
JSR-303: Bean Validation
JSR-303 - Bean Validation is a specification to standardize the validation of Java beans through annotations. Instead of creating validation rules in separate classes, JSR-303 promoting that validation rules to be specified directly in the code they are intended to validate, by using annotations directly in a Java bean class.
Using springfox-bean-validators
In previous article, we learn about Spring Boot 2 RESTful API Documentation With Swagger 2 using SpringFox library (SpringFox 2.9.2
). SpringFox can generate Swagger documentation based on JSR-303 (Bean Validation) annotations automatically, so we can utilize what we already have in our code without writing another annotations for API documentation manually and separately. In SpringFox version greater than 2.3.2, support for bean validation annotations was added, specifically for @NotNull, @Min, @Max, and @Size.
To enable this feature in our project, we need to add the springfox-bean-validators
dependency :
<dependency> <groupId>io.springfox</groupId> <artifactId>springfox-bean-validators</artifactId> <version>2.9.2</version> </dependency>
And then, we need to import the configuration from the springfox-bean-validators
module as shown below:
package com.dariawan.contactapp.config;
...
import springfox.bean.validators.configuration.BeanValidatorPluginsConfiguration;
...
@Configuration
@EnableSwagger2
@Import(BeanValidatorPluginsConfiguration.class)
public class SwaggerConfig {
...
}
}
Now, we complete the validation in Contact bean, final code as follow:
package com.dariawan.contactapp.domain;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.validation.constraints.Email;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import lombok.Getter;
import lombok.Setter;
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
@ApiModel(description = "Class representing a contact in the application.")
@Entity
@Table(name = "contact")
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
@Getter
@Setter
public class Contact implements Serializable {
private static final long serialVersionUID = 4048798961366546485L;
@ApiModelProperty(notes = "Unique identifier of the Contact.",
example = "1", required = true, position = 0)
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private Long id;
@ApiModelProperty(notes = "Name of the contact.",
example = "Jessica Abigail", required = true, position = 1)
@NotBlank
@Size(max = 100)
private String name;
@ApiModelProperty(notes = "Phone number of the contact.",
example = "62482211", required = false, position = 2)
@Pattern(regexp ="^\\+?[0-9. ()-]{7,25}$", message = "Phone number")
@Size(max = 25)
private String phone;
@ApiModelProperty(notes = "Email address of the contact.",
example = "[email protected]", required = false, position = 3)
@Email(message = "Email Address")
@Size(max = 100)
private String email;
@ApiModelProperty(notes = "Address line 1 of the contact.",
example = "888 Constantine Ave, #54", required = false, position = 4)
@Size(max = 50)
private String address1;
@ApiModelProperty(notes = "Address line 2 of the contact.",
example = "San Angeles", required = false, position = 5)
@Size(max = 50)
private String address2;
@ApiModelProperty(notes = "Address line 3 of the contact.",
example = "Florida", required = false, position = 6)
@Size(max = 50)
private String address3;
@ApiModelProperty(notes = "Postal code of the contact.",
example = "32106", required = false, position = 7)
@Size(max = 20)
private String postalCode;
@ApiModelProperty(notes = "Notes about the contact.",
example = "Meet her at Spring Boot Conference", required = false, position = 8)
@Column(length = 4000)
private String note;
}
Here the documentation of Contact model without using springfox-bean-validators
:
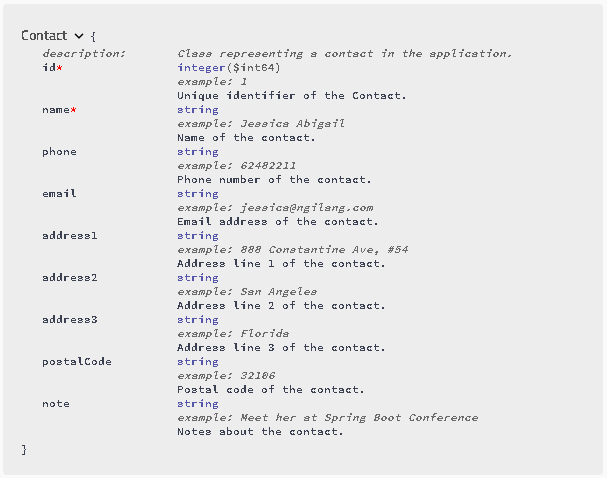
Without springfox-bean-validators
And here after include and use springfox-bean-validators
:
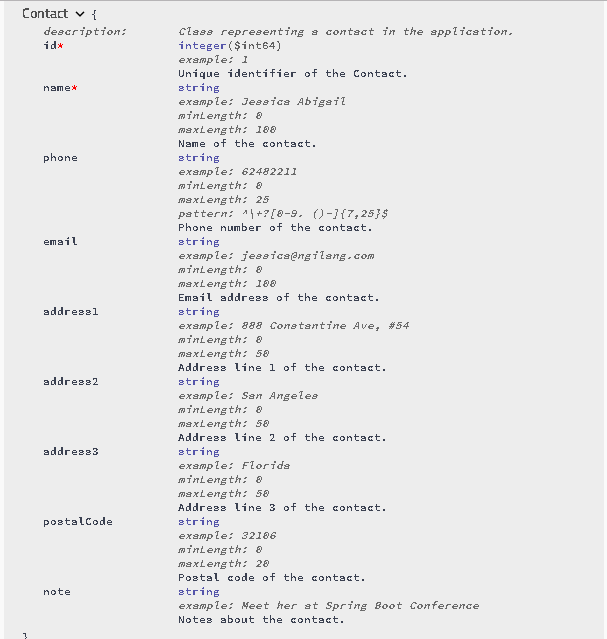
With springfox-bean-validators