Association, Aggregation, and Composition in Java
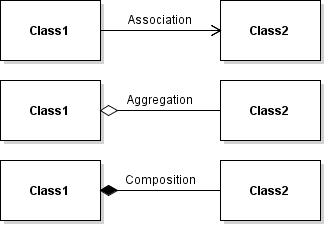
Association, Aggregation, and Composition
Association
Association is a relationship between two separate classes that establishes through their objects. Each objects have their own life-cycle and there is no owner. Association can be one-to-one, one-to-many, many-to-one, many-to-many.
Let's take an example of Teacher and Student. Multiple students can associate with single teacher and single student can associate with multiple teachers, but there is no ownership between the objects and both have their own life-cycle. Both can be created and deleted independently.
import java.util.ArrayList;
import java.util.List;
public class Teacher {
private final String name;
private final List<Student> students = new ArrayList<>();
// teacher name
Teacher(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public void addStudent(Student student) {
student.addTeacher(this);
this.students.add(student);
}
public List<Student> getStudents() {
return students;
}
public void print() {
System.out.println("Teacher " + this.name + "'s students are:");
for (Student student:this.students) {
System.out.println("- " + student.getName());
}
}
}
import java.util.ArrayList;
import java.util.List;
public class Student {
private final String name;
private final List<Teacher> teachers = new ArrayList<>();
// student name
Student(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public void addTeacher(Teacher teacher) {
this.teachers.add(teacher);
}
public List<Teacher> getTeachers() {
return teachers;
}
public void print() {
System.out.println("Student " + this.name + "'s teachers are:");
for (Teacher teacher:this.teachers) {
System.out.println("- " + teacher.getName());
}
}
}
public class Association {
public static void main(String[] args) {
Teacher teacher1 = new Teacher("Onizuka");
Teacher teacher2 = new Teacher("Fuyutsuki");
Student student1 = new Student("Nomura");
Student student2 = new Student("Aizawa");
Student student3 = new Student("Yoshikawa");
Student student4 = new Student("Uehara");
teacher1.addStudent(student1);
teacher1.addStudent(student2);
teacher1.addStudent(student3);
teacher2.addStudent(student2);
teacher2.addStudent(student3);
teacher2.addStudent(student4);
teacher1.print();
teacher2.print();
student1.print();
student2.print();
student3.print();
student4.print();
}
}
/*
Output:
------
Teacher Onizuka's students are:
- Nomura
- Aizawa
- Yoshikawa
Teacher Fuyutsuki's students are:
- Aizawa
- Yoshikawa
- Uehara
Student Nomura's teachers are:
- Onizuka
Student Aizawa's teachers are:
- Onizuka
- Fuyutsuki
Student Yoshikawa's teachers are:
- Onizuka
- Fuyutsuki
Student Uehara's teachers are:
- Fuyutsuki
*/
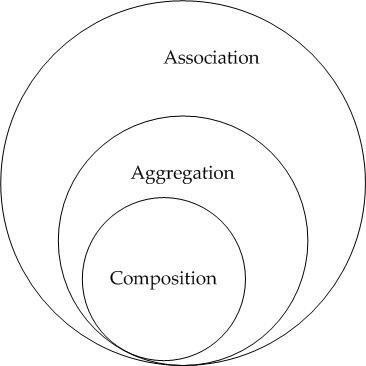
Association
Aggregation and Composition are subsets of association meaning they are specific cases of association.
Aggregation
Aggregation is a specialized form of Association where all objects have their own life cycle, where the child can exist independently of the parent. Aggregation is also called a “Has-a” relationship.
Let's take an example of Supervisor and Subordinate. An employee (as a subordinate) can not belong to multiple supervisors, but if we delete the supervisor, the employee object (subordinate) will not be destroyed. We can think about it as a “has-a” relationship.
import java.util.ArrayList;
import java.util.List;
public class Employee {
private final String name;
private Employee supervisor;
private final List<Employee> subordinates = new ArrayList<>();
// teacher name
Employee(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public Employee getSupervisor() {
return supervisor;
}
public void setSupervisor(Employee supervisor) {
this.supervisor = supervisor;
supervisor.subordinates.add(this);
}
public void print() {
System.out.println("Employee " + this.name + "'s supervisor is:" +
(this.supervisor==null?"N.A.":supervisor.getName()));
System.out.println("Employee " + this.name + "'s subordinates are:");
for (Employee employee:this.subordinates) {
System.out.println("- " + employee.getName());
}
}
}
public class Aggregation {
public static void main(String[] args) {
Employee employee1 = new Employee("Systrom");
Employee employee2 = new Employee("Krieger");
Employee employee3 = new Employee("Riedel");
Employee employee4 = new Employee("Sweeney");
Employee employee5 = new Employee("Zollman");
Employee employee6 = new Employee("Cole");
Employee employee7 = new Employee("Hochmuth");
Employee employee8 = new Employee("McAllister");
employee3.setSupervisor(employee1);
employee4.setSupervisor(employee1);
employee5.setSupervisor(employee1);
employee6.setSupervisor(employee2);
employee7.setSupervisor(employee2);
employee8.setSupervisor(employee2);
employee1.print();
employee2.print();
employee3.print();
employee8.print();
}
}
/*
Output:
------
Employee Systrom's supervisor is:N.A.
Employee Systrom's subordinates are:
- Riedel
- Sweeney
- Zollman
Employee Krieger's supervisor is:N.A.
Employee Krieger's subordinates are:
- Cole
- Hochmuth
- McAllister
Employee Riedel's supervisor is:Systrom
Employee Riedel's subordinates are:
Employee McAllister's supervisor is:Krieger
Employee McAllister's subordinates are:
*/
In above example, we use Employee class as type of Supervisor and Subordinate. The relationship is established between objects (class instances). Feel free to create specialized class Supervisor for supervisor.
Composition
Composition is specialized form of Aggregation and we can call this as a “death” relationship. Child object does not have its life-cycle and if parent object is deleted, all child objects will also be deleted.
Let's take an example of Car and Engine. Car is the owner of engine, engine is created when Car is created, and it's destroyed when Car is destroyed.
public enum EngineType {
PETROL,
DIESEL
}
public class Engine {
private final EngineType engineType;
public EngineType getEngineType() {
return engineType;
}
public Engine(EngineType engineType) {
this.engineType = engineType;
}
}
public class Car {
private final String name;
private final Engine engine;
public String getName() {
return name;
}
public Engine getEngine() {
return engine;
}
public Car(String name, Engine engine) {
this.name = name;
this.engine = engine;
}
public void print() {
System.out.println("Car " + this.name +
" engine is " + this.engine.getEngineType().toString());
}
}
public class Composition {
public static void main(String[] args) {
Car car1 = new Car("Peugeot 3008", new Engine(EngineType.PETROL));
Car car2 = new Car("BMW X5 Diesel", new Engine(EngineType.DIESEL));
car1.print();
car2.print();
}
}
/*
Output:
------
Car Peugeot 3008 engine is PETROL
Car BMW X5 Diesel engine is DIESEL
*/
Summary
Let's check below table for association, aggregation and composition brief summary:
Association | Aggregation | Composition | |
---|---|---|---|
Related to Association | Special type of Association. | Special type of Aggregation | |
Weak association | Strong association | ||
Relation | Has-A | Owns | |
one object is the owner of another object. | one object is contained in another object | ||
Owner | No owner | Single owner | Single owner |
Life-cycle | own life-cycle | own life-cycle | owner life-cycle |
Child object | independent | belong to single parent | belong to single parent |