Object-Oriented Programming Concepts
If you start programming in Java, you must familiar with Object Oriented Programming (OOP) Concept. Without knowledge about OOP concepts, you will not be able (elegantly) to design systems in object oriented programming model.
Core Object Oriented Programming Concepts are:
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
And object relation concepts:
- Association
- Aggregation
- Composition
Let’s look into these concepts one by one:
Inheritance
Inheritance is a mechanism where a new class is derived from an existing class. In Java, classes can inherit the methods and properties of another class.
A class that derived from another class is called a subclass (or child class), and the class from which a subclass is derived is called a superclass (or parent class / base class). A subclass can have only one superclass, but a superclass may have one or more subclasses.
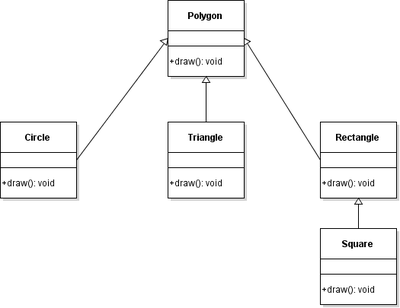
Inheritance and Polymorphism
More in Inheritance in Java
Polymorphism
Polymorphism refers to a programming language's ability to process objects differently depending on their data type or class. As example, in Java you have a superclass with method print() which is inherited by subclasses. But in subclasses, the method print() you want something different from method print() in superclass. You want the ability to redefine methods for subclasses, this is what we call polymorphism.
There are two types of polymorphism in Java: compile-time polymorphism (where we can perform method overloading) and runtime polymorphism (where we perform method overriding).
More in Polymorphism in Java
Encapsulation
Encapsulation is the process of hiding information details and protecting data and behavior of an object from misuse by other objects. In Java, encapsulation is done using classes, interfaces, access modifiers (public, protected, private, and "default"), setters and getters.
More in Encapsulation in Java
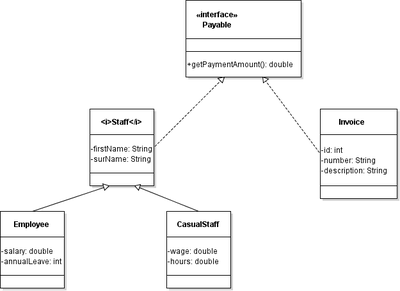
Payable (Interface) and Staff (Abstract)
Abstraction
Abstraction is a process of hiding the implementation details from the user, and showing only the essential things to the user. In Java, you can achieve abstraction in two ways: abstract classes and interfaces.
More in Abstraction in Java.
Association
In association, relation between two separate classes establishes through their objects. Association can be one-to-one, one-to-many, many-to-one, many-to-many.
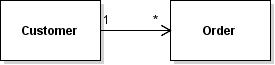
Association
Let’s take an example of Customer and Order. One customer can associate with multiple orders. It's represent one-to-many relationship.
Aggregation and Composition are two subsets of association, each of them represent specific case of association:
Aggregation
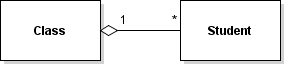
Aggregation
Aggregation is a specialized form of Association where all objects have their own life cycle, where the child can exist independently of the parent. Aggregation is also called a “Has-a” relationship.
Let’s take an example of Class (parent) and Student (child). Delete the Class, Student objects will not be destroyed and still exists.
Composition
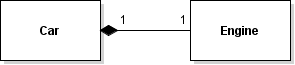
Composition
Composition is a restricted form of Aggregation in which two classes are highly dependent on each other. There's a strong life-cycle dependency between the parent and child. The parent cannot exist without the child, and the child has no meaning without the parent.
Let’s take an example of Car (parent) and Engine (child). A car needs engine to run. Although engine may exist as separate parts, detached from a specific car, but it's no use without the parent (car).