Spring Boot Quick Start
Spring Boot is a Framework to ease the bootstrapping and development of new Spring Applications. It's offers a fast way to build applications, that seems like magic. The magic that is applied by Spring Boot is as follows:
- Automatic configuration:Spring Boot automatically takes care of the application configuration. Most application have a common configuration pattern that automatically provided, with options for overriding the configuration if needed. Check Spring Boot Auto Configuration.
- Starters: Starters provide the required dependencies needed for the build according to the type of project chosen. There are many starters available, for Web, Data JPA, Test, etc. Check Spring Boot Starter for more details.
- Developer Tools: Spring Boot includes an additional set of tools to enhance the convenience of application development, like to automatically restarts a running application as soon as any change is made in the files situated in the classpath. Refer to Spring Boot Developer Tools.
With Spring Boot, it's easy to create standalone applications with embedded Tomcat/Jetty/Undertow. Spring Boot also provides metrics, health checks, and externalized configuration.
Without further ado, let's quickly create our Spring Boot application!
Scaffolding Spring Boot Application
There are two ways to quickly bootstrap your Spring boot application -
- Using Spring Initializr hosted at https://start.spring.io/
- Using Spring Boot CLI
Using Spring Initializr
Spring Initializr is the easiest option to generate Spring project with necessary configuration by using user friendly web interface.
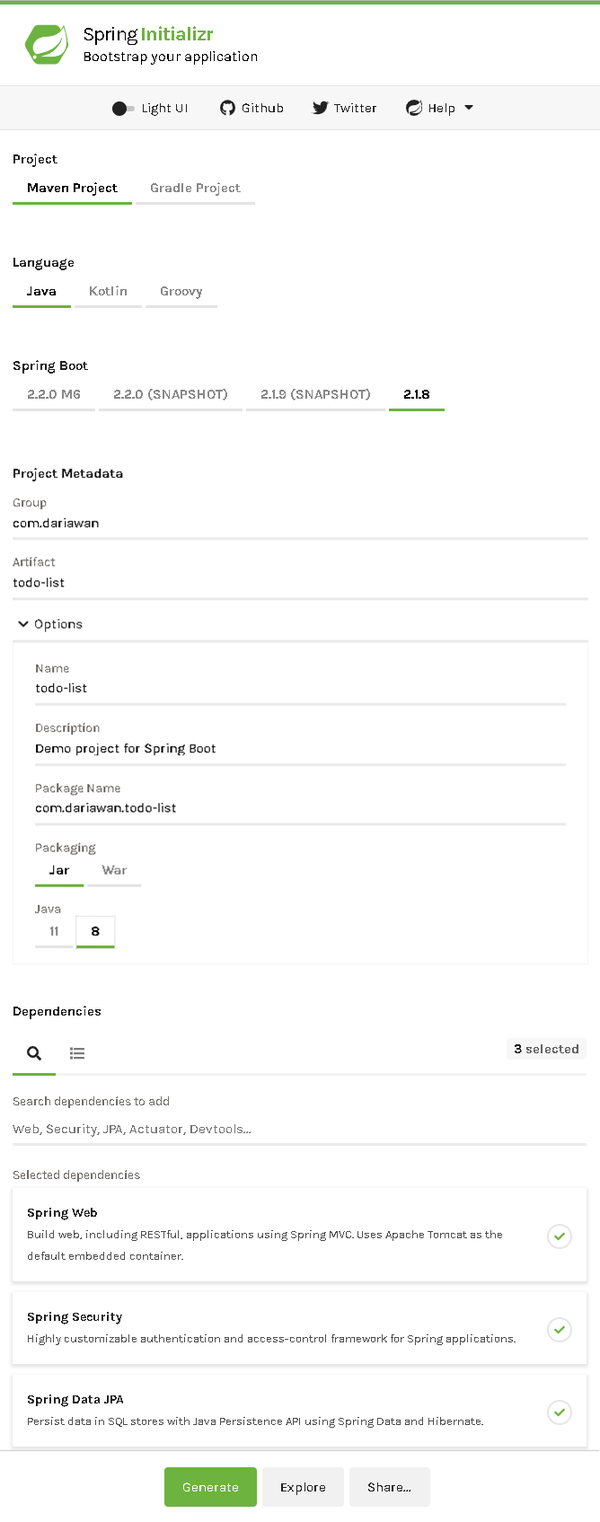
Spring Initializr - Bootstrap your application
Here steps to use Spring Initializr online web application:
- Go to https://start.spring.io/
- Select project type: Maven Project or Gradle Project (default: Maven Project)
- Select programming language: Java, Kotlin, or Groovy (default: Java)
- Choose Spring Boot version - this is depends on the available versions available at that time
- Enter Project Metadata: Group and Artifact
- Project Metadata's Options: Name, Description, Package Name, Packaging (jar or war), and Java (version 11 or 8). Default is jar with Java 8.
- Add Dependencies. We can search and add multiple dependencies, as example: Spring Web, Spring Security, Spring Data JPA, etc
- Click Generate to generate your project.
The tool will generate and produce a zip file containing project’s prototype. The generated prototype will have pom.xml (if using maven) or build.gradle (if using gradle), all the project directories with all the dependencies that we already selected. Unzip this file and import it in an IDE of your choice to start work with our application.
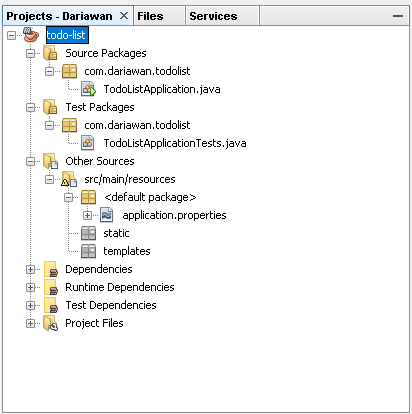
todo-list project
Since in this example we are generating a maven project, here the pom.xml generated (and enhanced):
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.8.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.dariawan</groupId>
<artifactId>todo-list</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>todo-list</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
- The
<parent>
tag tells Maven to inherit the properties ofspring-boot-starter-parent
, such configs, dependencies, etc. Refer to Spring Boot Starter Parent for details. - The
<dependencies>
tag contains all the project dependencies. Please notes that Spring Boot Starter Parent will manages default versions of artifacts (including plugins). - The
<build>
contains build plugins, such asspring-boot-maven-plugin
.
We have several dependencies: spring-boot-starter-web
, spring-boot-starter-data-jpa
, and spring-boot-starter-security
. Because we are using Spring Data JPA, we need to configure datasource. In our sample initialization above, we doesn't add any datasource dependency. It's okay, that doesn't mean that we need to re-do Spring Initializr. As told, this is just another Spring project. Just add dependency for MySQL Connector to use (or connect) to MySQL database:
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency>
And amend application.properties to facilitate datasource:
# Connection url for the database "todo"
spring.datasource.url = jdbc:mysql://localhost:3306/todo?serverTimezone=Asia/Singapore
# Username and password
spring.datasource.username = barista
spring.datasource.password = cappuccino
# Allows Hibernate to generate SQL optimized for a particular DBMS
spring.jpa.hibernate.dialect = org.hibernate.dialect.MySQL5Dialect
spring.jpa.hibernate.naming_strategy = org.hibernate.cfg.ImprovedNamingStrategy
And we're set! Run our application from IDE, or from terminal: go to the project’s root directory and type following command:
$ mvn spring-boot:run
Here the result:
[INFO] Scanning for projects... [INFO] [INFO] -----------------------< com.dariawan:todo-list >----------------------- [INFO] Building todo-list 0.0.1-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] >>> spring-boot-maven-plugin:2.1.8.RELEASE:run (default-cli) > test-compile @ todo-list >>> [INFO] [INFO] --- maven-resources-plugin:3.1.0:resources (default-resources) @ todo-list --- [INFO] Using 'UTF-8' encoding to copy filtered resources. [INFO] Copying 1 resource [INFO] Copying 0 resource [INFO] [INFO] --- maven-compiler-plugin:3.8.1:compile (default-compile) @ todo-list --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] --- maven-resources-plugin:3.1.0:testResources (default-testResources) @ todo-list --- [INFO] Using 'UTF-8' encoding to copy filtered resources. [INFO] skip non existing resourceDirectory D:\Projects\github\todo-list\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.1:testCompile (default-testCompile) @ todo-list --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] <<< spring-boot-maven-plugin:2.1.8.RELEASE:run (default-cli) < test-compile @ todo-list <<< [INFO] [INFO] [INFO] --- spring-boot-maven-plugin:2.1.8.RELEASE:run (default-cli) @ todo-list --- . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v2.1.8.RELEASE) 2019-10-01 00:44:24.541 INFO 8788 --- [ main] c.dariawan.todolist.TodoListApplication : Starting TodoListApplication on DessonAr with PID 8788 (D:\Projects\github\todo-list\target\classes started by Desson in D:\Projects\github\todo-list) 2019-10-01 00:44:24.546 INFO 8788 --- [ main] c.dariawan.todolist.TodoListApplication : No active profile set, falling back to default profiles: default 2019-10-01 00:44:25.585 INFO 8788 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Bootstrapping Spring Data repositories in DEFAULT mode. 2019-10-01 00:44:25.614 INFO 8788 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Finished Spring Data repository scanning in 19ms. Found 0 repository interfaces. 2019-10-01 00:44:26.096 INFO 8788 --- [ main] trationDelegate$BeanPostProcessorChecker : Bean 'org.springframework.transaction.annotation.ProxyTransactionManagementConfiguration' of type [org.springframework.transaction.annotation.ProxyTransactionManagementConfiguration$$EnhancerBySpringCGLIB$$c6492e32] is not eligible for getting processed by all BeanPostProcessors (for example: not eligible for auto-proxying) 2019-10-01 00:44:26.769 INFO 8788 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http) 2019-10-01 00:44:26.811 INFO 8788 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat] 2019-10-01 00:44:26.811 INFO 8788 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.24] 2019-10-01 00:44:26.952 INFO 8788 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext 2019-10-01 00:44:26.953 INFO 8788 --- [ main] o.s.web.context.ContextLoader : Root WebApplicationContext: initialization completed in 2349 ms 2019-10-01 00:44:27.241 INFO 8788 --- [ main] com.zaxxer.hikari.HikariDataSource : HikariPool-1 - Starting... 2019-10-01 00:44:27.444 INFO 8788 --- [ main] com.zaxxer.hikari.HikariDataSource : HikariPool-1 - Start completed. 2019-10-01 00:44:27.523 INFO 8788 --- [ main] o.hibernate.jpa.internal.util.LogHelper : HHH000204: Processing PersistenceUnitInfo [ name: default ...] 2019-10-01 00:44:27.634 INFO 8788 --- [ main] org.hibernate.Version : HHH000412: Hibernate Core {5.3.11.Final} 2019-10-01 00:44:27.637 INFO 8788 --- [ main] org.hibernate.cfg.Environment : HHH000206: hibernate.properties not found 2019-10-01 00:44:27.830 INFO 8788 --- [ main] o.hibernate.annotations.common.Version : HCANN000001: Hibernate Commons Annotations {5.0.4.Final} 2019-10-01 00:44:28.013 INFO 8788 --- [ main] org.hibernate.dialect.Dialect : HHH000400: Using dialect: org.hibernate.dialect.MySQL5Dialect 2019-10-01 00:44:28.274 INFO 8788 --- [ main] j.LocalContainerEntityManagerFactoryBean : Initialized JPA EntityManagerFactory for persistence unit 'default' 2019-10-01 00:44:28.803 INFO 8788 --- [ main] o.s.s.concurrent.ThreadPoolTaskExecutor : Initializing ExecutorService 'applicationTaskExecutor' 2019-10-01 00:44:28.872 WARN 8788 --- [ main] aWebConfiguration$JpaWebMvcConfiguration : spring.jpa.open-in-view is enabled by default. Therefore, database queries may be performed during view rendering. Explicitly configure spring.jpa.open-in-view to disable this warning 2019-10-01 00:44:29.265 INFO 8788 --- [ main] .s.s.UserDetailsServiceAutoConfiguration : Using generated security password: 604d13b2-97a8-491e-9099-5d82d285b940 2019-10-01 00:44:29.430 INFO 8788 --- [ main] o.s.s.web.DefaultSecurityFilterChain : Creating filter chain: any request, [org.springframework.security.web.context.request.async.WebAsyncManagerIntegrationFilter@52f6b7be, org.springframework.security.web.context.SecurityContextPersistenceFilter@7de6b8a5, org.springframework.security.web.header.HeaderWriterFilter@547342aa, org.springframework.security.web.csrf.CsrfFilter@78ee3a82, org.springframework.security.web.authentication.logout.LogoutFilter@6de6117a, org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter@7bb6bc7, org.springframework.security.web.authentication.ui.DefaultLoginPageGeneratingFilter@4821e6ad, org.springframework.security.web.authentication.ui.DefaultLogoutPageGeneratingFilter@7ad1f9e8, org.springframework.security.web.authentication.www.BasicAuthenticationFilter@2931c08c, org.springframework.security.web.savedrequest.RequestCacheAwareFilter@5b370b33, org.springframework.security.web.servletapi.SecurityContextHolderAwareRequestFilter@461f6611, org.springframework.security.web.authentication.AnonymousAuthenticationFilter@61d8deb5, org.springframework.security.web.session.SessionManagementFilter@b6383, org.springframework.security.web.access.ExceptionTranslationFilter@292dc8d2, org.springframework.security.web.access.intercept.FilterSecurityInterceptor@439abf50] 2019-10-01 00:44:29.560 INFO 8788 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path '' 2019-10-01 00:44:29.565 INFO 8788 --- [ main] c.dariawan.todolist.TodoListApplication : Started TodoListApplication in 5.56 seconds (JVM running for 10.405)
Application starts running without any error. Let's check several things that Spring Boot set for us:
- Since no active profile set, Spring falling back to default profiles. Profiles are used to configure application for different environments like dev, sit, uat, prod, ... you name it.
- Since we are using Spring Data JPA, Spring help to bootstrapping Spring Data repositories
- Spring initialize Tomcat on port 8080. Tomcat is default embedded server configured in Spring Boot.
- Spring Initialize embedded WebApplicationContext
- Hikari Pool started
- And so on... until application fully started
Yes, the are a lot happening when Spring starts, but Spring Boot ease the development/configuration process and hides the complexity from end developer.
Using Spring Boot CLI
The Spring Boot CLI (Command Line Interface) is a command line tool that you can use to quickly prototype with Spring. It lets you run Groovy scripts, which means that you have a familiar Java-like syntax without so much boilerplate code.
You do not need to use the CLI to work with Spring Boot, but it is definitely the quickest way to get a Spring application off the ground. Checkout on how to Installing the Spring Boot CLI. After installed, let's check the version of installed CLI:
$ spring --version Spring CLI v2.2.0.BUILD-SNAPSHOT
Here list of things that we can do with Spring Boot CLI:
$ spring --help usage: spring [--help] [--version] <command> [<args>] Available commands are: run [options] <files> [--] [args] Run a spring groovy script grab Download a spring groovy script's dependencies to ./repository jar [options] <jar-name> <files> Create a self-contained executable jar file from a Spring Groovy script war [options] <war-name> <files> Create a self-contained executable war file from a Spring Groovy script install [options] <coordinates> Install dependencies to the lib/ext directory uninstall [options] <coordinates> Uninstall dependencies from the lib/ext directory init [options] [location] Initialize a new project using Spring Initializr (start.spring.io) encodepassword [options] <password to encode> Encode a password for use with Spring Security shell Start a nested shell Common options: --debug Verbose mode Print additional status information for the command you are running See 'spring help <command>' for more information on a specific command.
We will focusing in spring init command to generate a new Spring Boot project.
$ spring help init spring init - Initialize a new project using Spring Initializr (start.spring.io) usage: spring init [options] [location] Option Description ------ ----------- -a, --artifactId <String> Project coordinates; infer archive name (for example 'test') -b, --boot-version <String> Spring Boot version (for example '1.2.0.RELEASE') --build <String> Build system to use (for example 'maven' or 'gradle') (default: maven) -d, --dependencies <String> Comma-separated list of dependency identifiers to include in the generated project --description <String> Project description -f, --force Force overwrite of existing files --format <String> Format of the generated content (for example 'build' for a build file, 'project' for a project archive) (default: project) -g, --groupId <String> Project coordinates (for example 'org.test') -j, --java-version <String> Language level (for example '1.8') -l, --language <String> Programming language (for example 'java') --list List the capabilities of the service. Use it to discover the dependencies and the types that are available -n, --name <String> Project name; infer application name -p, --packaging <String> Project packaging (for example 'jar') --package-name <String> Package name -t, --type <String> Project type. Not normally needed if you use -- build and/or --format. Check the capabilities of the service (--list) for more details --target <String> URL of the service to use (default: https://start. spring.io) -v, --version <String> Project version (for example '0.0.1-SNAPSHOT') -x, --extract Extract the project archive. Inferred if a location is specified without an extension examples: To list all the capabilities of the service: $ spring init --list To creates a default project: $ spring init To create a web my-app.zip: $ spring init -d=web my-app.zip To create a web/data-jpa gradle project unpacked: $ spring init -d=web,jpa --build=gradle my-dir
Here an example to generate a maven web application with JDBC, PostgreSQL and Thymeleaf dependencies
D:\Projects>spring init --name=meeting-dashboard --dependencies=web,data-jdbc,postgresql,devtools,thymeleaf --package-name=com.dariawan.meetdash meeting-dashboard Using service at https://start.spring.io Project extracted to 'D:\Projects\meeting-dashboard'
Similar like using Spring Initializr, import the resulted project to your IDE, and start working from there. As simple as that.
Let's create new java controller file: PingController
package com.dariawan.meetdash.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class PingController {
@GetMapping("/ping")
@ResponseBody
public String pong() {
return "pong :: Dariawan's Spring Boot";
}
}
Run mvn spring-boot:run, and after complete start, use browser to check http://localhost:8080/ping
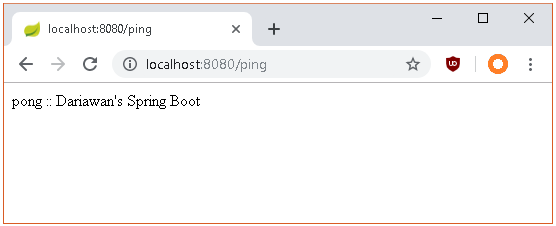
http://localhost:8080/ping
Conclusion
Spring Boot is simply Spring Framework + Embedded HTTP Servers (Tomcat/Jetty etc.) with "easy" configurations. In this article we learn about Spring Initializr and Spring Boot CLI to bootstrap a Spring Boot Application quickly. This framework enable us to leverage existing Spring knowledge and experience with faster pace.